Program:
#include<stdio.h>
#include<conio.h>
void main()
{
char c,number=0,ucharacter=0,lcharacter=0,space=0,symbol=0;
clrscr();
printf("\n enter the character \n\n");
do{
c=getchar();
if(c>='a'&&c<='z')
lcharacter++;
else
if(c>='A'&&c<='Z')
ucharacter++;
else
if(c==' ')
space++;
else
if(c>='0'&&c<='9')
number++;
elsesymbol++;
}
while(c!='\n');
printf("\nupper letter=%d\nlower letter=%d\nnumber=%d\nspace=%d\nsymbol=%d",ucharacter,lcharacter,number,space,(symbol-1));
getch();
}
Output:
#include<stdio.h>
#include<conio.h>
void main()
{
char c,number=0,ucharacter=0,lcharacter=0,space=0,symbol=0;
clrscr();
printf("\n enter the character \n\n");
do{
c=getchar();
if(c>='a'&&c<='z')
lcharacter++;
else
if(c>='A'&&c<='Z')
ucharacter++;
else
if(c==' ')
space++;
else
if(c>='0'&&c<='9')
number++;
elsesymbol++;
}
while(c!='\n');
printf("\nupper letter=%d\nlower letter=%d\nnumber=%d\nspace=%d\nsymbol=%d",ucharacter,lcharacter,number,space,(symbol-1));
getch();
}
Output:
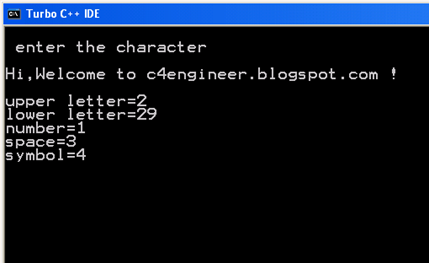